
Home Company Ordering 4Webcheck Utilities Support Buy

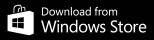
Face detection database app
/*global requestJSON*/
import { DynamoDBClient } from "@aws-sdk/client-dynamodb";
import {
DynamoDBDocumentClient,
PutCommand,
GetCommand,
} from "@aws-sdk/lib-dynamodb";
export const handler = async (event) => {
var name=event.queryStringParameters.name;
if (!name.match(/^(abc|xyz|totals|reset)$/))
{
const response = {
statusCode: 200,
body: 'Unknown'
};
return response;
}
let mybody;
// Create the DynamoDB service object
const client = new DynamoDBClient({});
const dynamo = DynamoDBDocumentClient.from(client);
const tableName = "mycounter";
if (name.match(/^(abc|xyz)$/))
{
var website;
if (name=='abc') website="abc.com";
if (name=='xyz') website="xyz.com";
mybody = await dynamo.send(
new GetCommand({
TableName:tableName,
Key:{"website":website},
}));
mybody=mybody.Item;
var visits=mybody.visits;
visits++;
var body = await dynamo.send(
new PutCommand({
TableName:tableName,
Item:{"website": website,
"visits": visits
},}));
}
if (name=='reset')
{
var thewebsites=["abc.com","xyz.com"];
for(website of thewebsites)
{
var body = await dynamo.send(
new PutCommand({
TableName:tableName,
Item:{"website": website,
"visits": 0
},}));
}
name='totals';
}
if (name=='totals')
{
var thewebsites=["abc.com","xyz.com"];
var thetotals=[];
for(website of thewebsites)
{
mybody = await dynamo.send(
new GetCommand({
TableName:tableName,
Key:{"website": website},
}));
mybody=mybody.Item;
thetotals.push(website+':'+mybody.visits);
}
const dataString = JSON.stringify(thetotals);
const response = {
statusCode: 200,
body: dataString
};
return response;
};
const response = {
statusCode: 200,
body: ''
};
return response;
};
Next click the Configuration tab and on the left Function URL and make it public so it can be called from a web page. Make a note of it.
{
"Version": "2024-04-16",
"Statement": [
{
"Effect": "Allow",
"Action": [
"dynamodb:GetItem",
"dynamodb:PutItem",
"dynamodb:UpdateItem"
],
"Resource": [
"arn:aws:dynamodb:thedynamoDB"
]
}
]
}
Back on the Lambda function, click Configuration and select Permissions on the left. For the Execicution role select DynamoDB_lambda
Finally, we need to deploy the function to make it available. Above the lambda code there is a Deploy option, just click it.
// For website abc.com
function myfunction() {
var req = new XMLHttpRequest();
req.open("GET", "Your_lambda_URL.aws/?name=abc");
req.send();
};
// For website xyz.com
function myfunction() {
var req = new XMLHttpRequest();
req.open("GET", "Your_lambda_URL.aws/?name=xyz");
req.send();
};
Finally in the Body tag, to run it when the page is loaded:
onload="javascript:myfunction()"
To view the totals from a browser, just use the lambda URL with the query string: